Evaluate, Deploy and Test the decision table
In this step, we use Java Code to evaluate the decision table. Then we deploy the web application to Apache Tomcat and verify the result in Cockpit.
Evaluate the Decision Table
To directly evaluate the decision table after deployment, add the following method to your Application class:
package org.camunda.bpm.getstarted.dmn;
@ProcessApplication("Dinner App DMN")
public class DinnerApplication extends ServletProcessApplication {
protected final static Logger LOGGER = Logger.getLogger(DinnerApplication.class.getName());
@PostDeploy
public void evaluateDecisionTable(ProcessEngine processEngine) {
DecisionService decisionService = processEngine.getDecisionService();
VariableMap variables = Variables.createVariables()
.putValue("season", "Spring")
.putValue("guestCount", 10);
DmnDecisionTableResult dishDecisionResult = decisionService.evaluateDecisionTableByKey("dish", variables);
String desiredDish = dishDecisionResult.getSingleEntry();
LOGGER.log(Level.INFO, "\n\nDesired dish: {0}\n\n", desiredDish);
}
}
Catch up: Get the Sources of Step-4.
Download as .zip or checkout the corresponding tag with Git.
You can checkout the current state from the GitHub repository.
If you have not cloned the repository yet, please execute the following command:
git clone https://github.com/camunda/camunda-get-started-dmn.git
To checkout the current state of the process application please execute the following command:
git checkout -f Step-4Or download as archive from here.
Build the Web Application with Maven
Select the pom.xml
in the Package Explorer, perform a right-click and select Run As / Maven Install
. This will generate a WAR file named dinner-dmn-0.1.0-SNAPSHOT.war
in the target/
folder of your Maven project.
Hint
If the dinner-dmn-0.1.0-SNAPSHOT.war
file is not visible after having performed the Maven build, you need to refresh the project (F5) in eclipse.
Deploy to Apache Tomcat
In order to deploy the process application, copy-paste the dinner-dmn-0.1.0-SNAPSHOT.war
from your Maven project to the $CAMUNDA_HOME/server/apache-tomcat/webapps
folder.
Check the log file of the Apache Tomcat server. If you see the following log message, the deployment was successful:
INFO org.camunda.commons.logging.BaseLogger.logInfo ENGINE-07015 Detected @ProcessApplication class 'org.camunda.bpm.getstarted.dish.DishApplication' INFO org.camunda.commons.logging.BaseLogger.logInfo ENGINE-08024 Found processes.xml file at ../webapps/dinner-dmn-0.1.0-SNAPSHOT/WEB-INF/classes/META-INF/processes.xml INFO org.camunda.commons.logging.BaseLogger.logInfo ENGINE-08023 Deployment summary for process archive 'dinner-dmn': dinnerDecisions.dmn INFO org.camunda.bpm.getstarted.dmn.DinnerApplication.evaluateDecisionTable Desired dish: Stew INFO org.camunda.commons.logging.BaseLogger.logInfo ENGINE-08050 Process application Dinner App DMN successfully deployed
Verify the Deployment with Cockpit
Now, use Cockpit to check if the decision table is successfully deployed. Go to http://localhost:8080/camunda/app/cockpit. Log in with demo / demo. Go to “Decisions” section. Your decision table Dish should be listed as deployed decision definition.
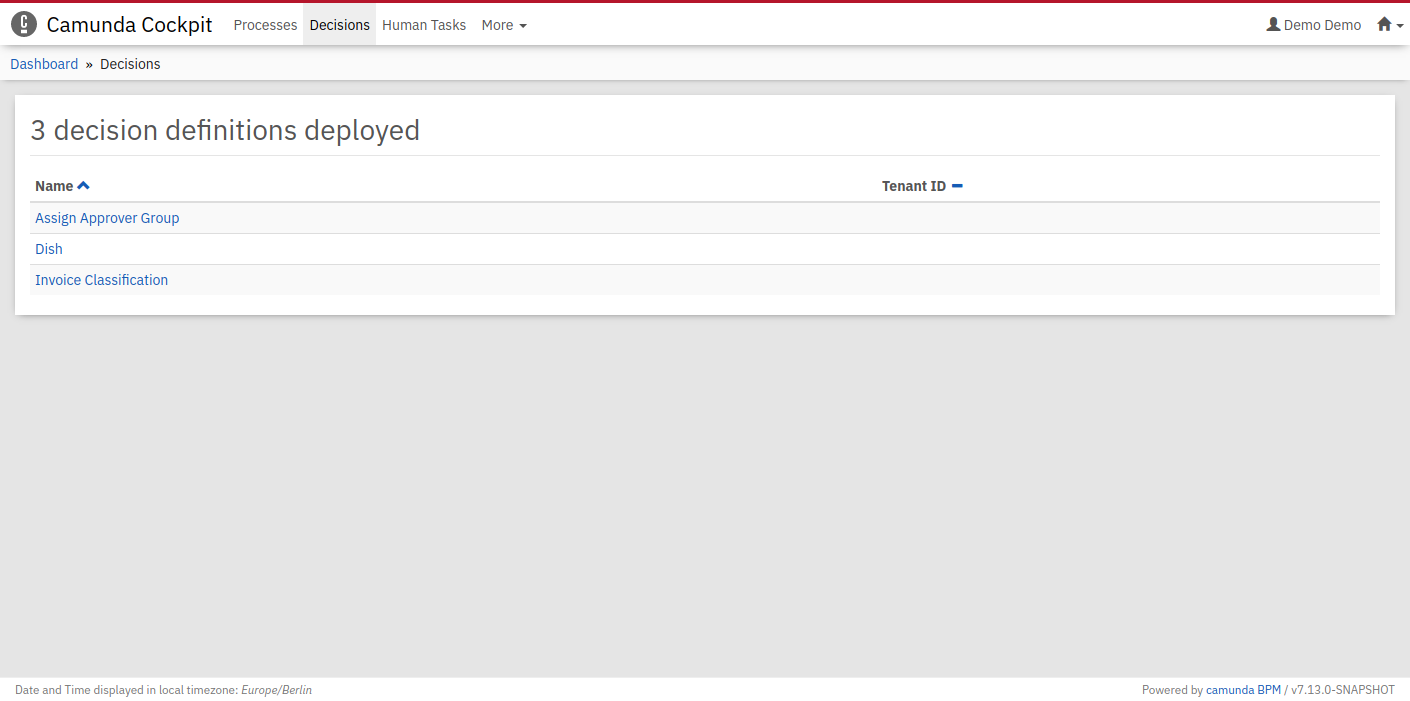
Verify the Evaluation with Cockpit
Click on the decision Dish. This opens a dialog where you see when the decision table was evaluated.
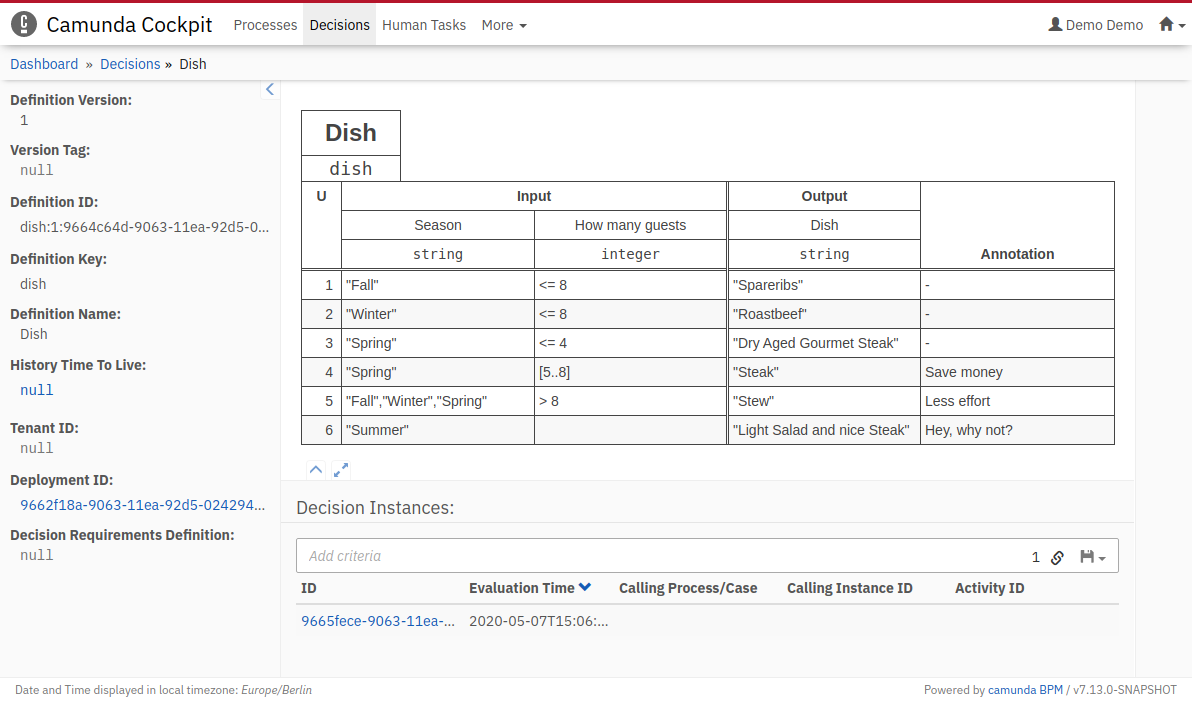
If you click on the id, you can see the historic data of the evaluation. The matched rules are highlighted and the input and output values are listed in the table below.
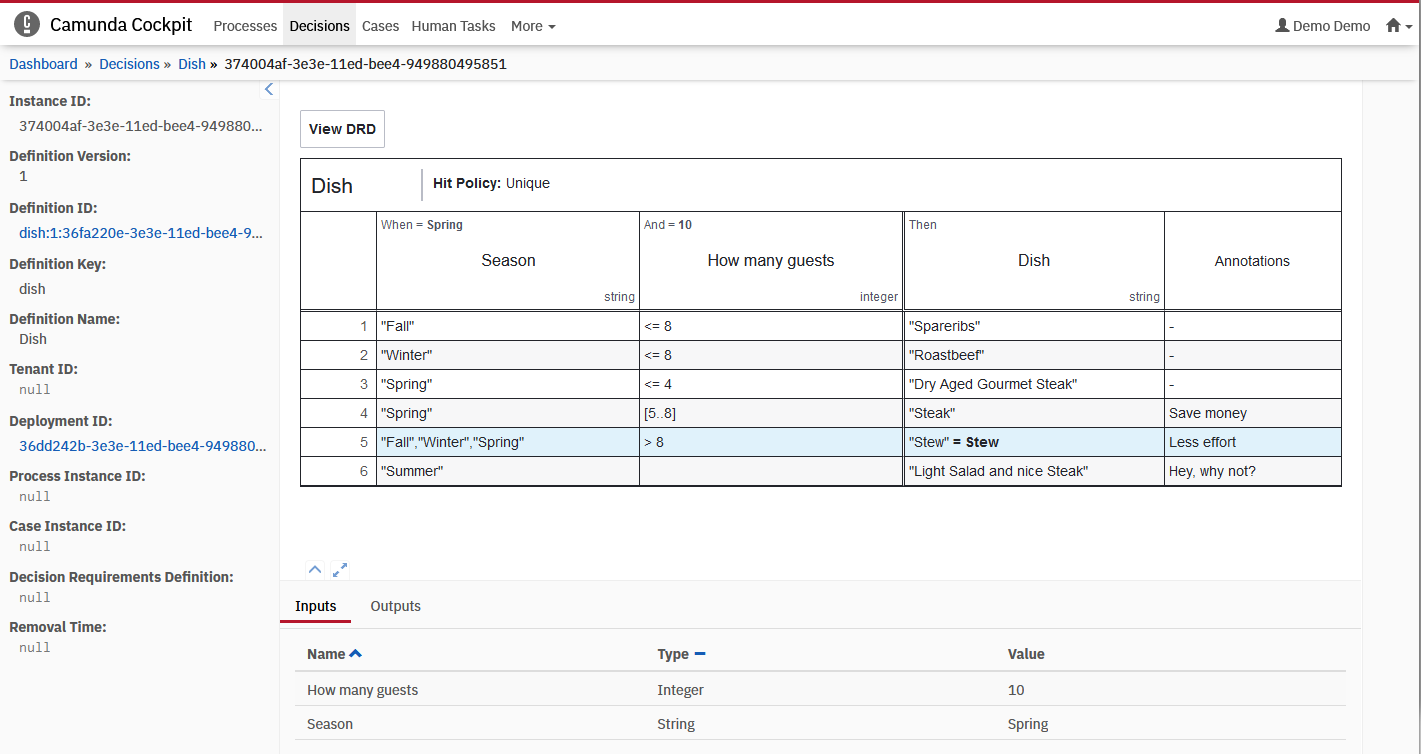
Verify that the 5th rule was matched and the output value for the desired dish is “Stew”.
Next Steps
Congratulations, you have now successfully set up a project with your first DMN decision table.
Next,
- see how you can evaluate the decision using the REST API,
- learn more about DMN by reading the DMN Reference,
- learn more about the Decision API exposed by Camunda Process Engine,
- check how you can invoke the decision from a BPMN Business Rule Task,
- Bonus Step: Decision Requirements Graph