Service Task
A Service Task is used to invoke services. In Camunda this is done by calling Java code or providing a work item for an external worker to complete asynchronously or invoking a logic which is implemented in form of webservices.
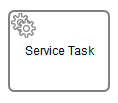
Calling Java Code
There are four ways of declaring how to invoke Java logic:
- Specifying a class that implements a JavaDelegate or ActivityBehavior
- Evaluating an expression that resolves to a delegation object
- Invoking a method expression
- Evaluating a value expression
To specify a class that is called during process execution, the fully qualified classname needs to be provided by the camunda:class
attribute.
<serviceTask id="javaService"
name="My Java Service Task"
camunda:class="org.camunda.bpm.MyJavaDelegate" />
Please refer to the Java Delegate section of the User Guide for details on how to implement a Java Delegate.
It is also possible to use an expression that resolves to an object. This object must follow the
same rules as objects that are created when the camunda:class
attribute is used.
<serviceTask id="beanService"
name="My Bean Service Task"
camunda:delegateExpression="${myDelegateBean}" />
Or an expression which calls a method or resolves to a value.
<serviceTask id="expressionService"
name="My Expression Service Task"
camunda:expression="${myBean.doWork()}" />
For more information about expression language as delegation code, please see the corresponding section of the User Guide.
It is also possible to invoke logic which is implemented in form of webservices. camunda:connector
is an extension that allows calling REST/SOAP APIs directly from the workflow. For more information about using connectors, please see the corresponding section of the User Guide
Generic Java Delegates & Field Injection
You can easily write generic Java Delegate classes which can be configured later on via the BPMN 2.0 XML in the Service Task. Please refer to the Field Injection section of the User Guide for details.
Service Task Results
The return value of a service execution (for a Service Task exclusively using expressions) can be assigned to an already existing or to a new process variable by specifying the process variable name as a literal value for the camunda:resultVariable
attribute of a Service Task definition. Any existing value for a specific process variable will be overwritten by the result value of the service execution. When not specifying a result variable name, the service execution result value is ignored.
<serviceTask id="aMethodExpressionServiceTask"
camunda:expression="#{myService.doSomething()}"
camunda:resultVariable="myVar" />
In the example above, the result of the service execution (the return value of the doSomething()
method invocation on object myService
) is set to the process variable named myVar
after the service execution completes.
Result variables and multi-instance
Note that when you use camunda:resultVariable
in a multi-instance construct, for example in a multi-instance subprocess, the result variable is overwritten every time the task completes, which may appear as random behavior. See camunda:resultVariable for details.
External Tasks
In contrast to calling Java code, where the process engine synchronously invokes Java logic, it is possible to implement a Service Task outside of the process engine’s boundaries in the form of an external task. When a Service Task is declared external, the process engine offers a work item to workers that independently poll the engine for work to do. This decouples the implementation of tasks from the process engine and allows to cross system and technology boundaries. See the user guide on external tasks for details on the concept and the relevant API.
To declare a Service Task to be handled externally, the attribute camunda:type
can be set to external
and the attribute camunda:topic
specifies the external task’s topic. For example, the following XML snippet defines an external Service Task with topic ShipmentProcessing
:
<serviceTask id="anExternalServiceTask"
camunda:type="external"
camunda:topic="ShipmentProcessing" />
Camunda Extensions
Attributes | camunda:asyncBefore, camunda:asyncAfter, camunda:class, camunda:delegateExpression, camunda:exclusive, camunda:expression, camunda:jobPriority, camunda:resultVariable, camunda:topic, camunda:type, camunda:taskPriority |
---|---|
Extension Elements | camunda:errorEventDefinition, camunda:failedJobRetryTimeCycle, camunda:field, camunda:connector, camunda:inputOutput |
Constraints |
One of the attributes camunda:class , camunda:delegateExpression ,
camunda:type or camunda:expression is mandatory
|
The attribute camunda:resultVariable can only be used in combination with the
camunda:expression attribute
|
|
The camunda:exclusive attribute is only evaluated if the attribute
camunda:asyncBefore or camunda:asyncAfter is set to true
|
|
The attribute camunda:topic can only be used when the camunda:type attribute is set to external .
|
|
The attribute camunda:taskPriority can only be used when the camunda:type attribute is set to external .
|
|
The element camunda:errorEventDefinition can only be used as a child of serviceTask when the camunda:type attribute is set to external .
|
Additional Resources
- Tasks in the BPMN Modeling Reference section
- How to call a Webservice from BPMN. Please note that this article is outdated. However, it is still valid regarding how you would call a Web Service using the process engine.