Script Task
A Script Task is an automated activity. When a process execution arrives at the Script Task, the corresponding script is executed.
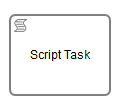
A Script Task is defined by specifying the script and the scriptFormat.
<scriptTask id="theScriptTask" name="Execute script" scriptFormat="groovy">
<script>
sum = 0
for ( i in inputArray ) {
sum += i
}
</script>
</scriptTask>
The value of the scriptFormat attribute must be a name that is compatible with JSR-223 (Scripting for the Java Platform). If you want to use a (JSR-223 compatible) scripting engine, you need to to add the corresponding jar to the classpath and use the appropriate name.
The script source code has to be added as the text content of the script
child element.
Alternatively, the source code can be specified as an expression or external resource. For more
information on the possible ways of providing the script source code please see the corresponding
section of the User Guide.
For general information about scripting in the process engine, please see the Scripting section of the User Guide.
Supported Script Languages
Camunda 7 should work with most of the JSR-223 compatible script engine implementations. We test integration for Groovy, JavaScript, JRuby and Jython. See the Third Party Dependencies section of the User Guide for more details.
Variables in Scripts
All process variables that are accessible through the execution that arrives in the Script Task can be used within the script. In the example below, the script variable inputArray
is in fact a process variable (an array of integers).
<script>
sum = 0
for ( i in inputArray ) {
sum += i
}
</script>
It’s also possible to set process variables in a script. Variables can be set by using the setVariable(...)
methods provided by the VariableScope
interface:
<script>
sum = 0
for ( i in inputArray ) {
sum += i
}
execution.setVariable("sum", sum);
</script>
Enabling auto-storing of Script Variables
By setting the property autoStoreScriptVariables
to true
in the process engine configuration, the process engine will automatically store all global script variables as process variables. This was the default behavior in Camunda 7.0 and 7.1 but it only reliably works for the Groovy scripting language (see the Set autoStoreScriptVariables section of the Migration Guide for more information).
To use this feature, you have to
- set
autoStoreScriptVariables
totrue
in the process engine configuration - prefix all script variables that should not be stored as script variables using the
def
keyword:def sum = 0
. In this case the variablesum
will not be stored as process variable.
Groovy-Support only
The configuration flag autoStoreScriptVariables
is only supported for Groovy Script Tasks. If enabled for other script languages,
it is not guaranteed which variables will be exported by the script engine. For
example, Ruby will not export any of the script variables at all.
Note: the following names are reserved and cannot be used as variable names:
out
, out:print
, lang:import
, context
, elcontext
.
Script Results
The return value of a Script Task can be assigned to a previously existing or to a new process variable by specifying the process variable name as a literal value for the camunda:resultVariable
attribute of a Script Task definition. Any existing value for a specific process variable will be overwritten by the result value of the script execution. When a result variable name is not specified, the script result value gets ignored.
<scriptTask id="theScriptTask" name="Execute script" scriptFormat="juel" camunda:resultVariable="myVar">
<script>#{echo}</script>
</scriptTask>
In the above example, the result of the script execution (the value of the resolved expression #{echo}
) is set to the process variable named myVar
after the script completes.
Result variables and multi-instance
Note that when you use camunda:resultVariable
in a multi-instance construct, for example in a multi-instance subprocess, the result variable is overwritten every time the task completes, which may appear as random behavior. See camunda:resultVariable for details.
Camunda Extensions
Attributes | camunda:asyncBefore, camunda:asyncAfter, camunda:exclusive, camunda:jobPriority, camunda:resultVariable, camunda:resource |
---|---|
Extension Elements | camunda:failedJobRetryTimeCycle, camunda:inputOutput |
Constraints |
The camunda:exclusive attribute is only evaluated if the attribute
camunda:asyncBefore or camunda:asyncAfter is set to true
|