Receive Task
A Receive Task is a simple task that waits for the arrival of a certain message. When the process execution arrives at a Receive Task, the process state is committed to the persistence storage. This means that the process will stay in this wait state until a specific message is received by the engine, which triggers continuation of the process beyond the Receive Task.
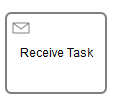
A Receive Task with a message reference can be triggered like an ordinary event:
<definitions ...>
<message id="newInvoice" name="newInvoiceMessage"/>
<process ...>
<receiveTask id="waitState" name="wait" messageRef="newInvoice">
...
You can then either correlate the message:
// correlate the message
runtimeService.createMessageCorrelation(subscription.getEventName())
.processInstanceBusinessKey("AB-123")
.correlate();
Or explicitly query for the subscription and trigger it:
ProcessInstance pi = runtimeService.startProcessInstanceByKey("processWaitingInReceiveTask");
EventSubscription subscription = runtimeService.createEventSubscriptionQuery()
.processInstanceId(pi.getId()).eventType("message").singleResult();
runtimeService.messageEventReceived(subscription.getEventName(), subscription.getExecutionId());
Correlation of a parallel multi instance isn’t possible because the subscription can’t be identified unambiguously.
To continue a process instance that is currently waiting at a Receive Task without a message reference, the runtimeService.signal(executionId)
can be called, using the id of the execution that arrived in the Receive Task.
<receiveTask id="waitState" name="wait" />
The following code snippet shows how this works in practice:
ProcessInstance pi = runtimeService.startProcessInstanceByKey("receiveTask");
Execution execution = runtimeService.createExecutionQuery()
.processInstanceId(pi.getId()).activityId("waitState").singleResult();
runtimeService.signal(execution.getId());
Camunda Extensions
Attributes | camunda:asyncBefore, camunda:asyncAfter, camunda:exclusive, camunda:jobPriority |
---|---|
Extension Elements | camunda:failedJobRetryTimeCycle, camunda:inputOutput |
Constraints |
The camunda:exclusive attribute is only evaluated if the attribute
camunda:asyncBefore or camunda:asyncAfter is set to true
|